Save Time Launching Projects on iTerm2 - Python Script
Running different terminals and navigating to folders one by one? Automate it with one script using iTerm2.
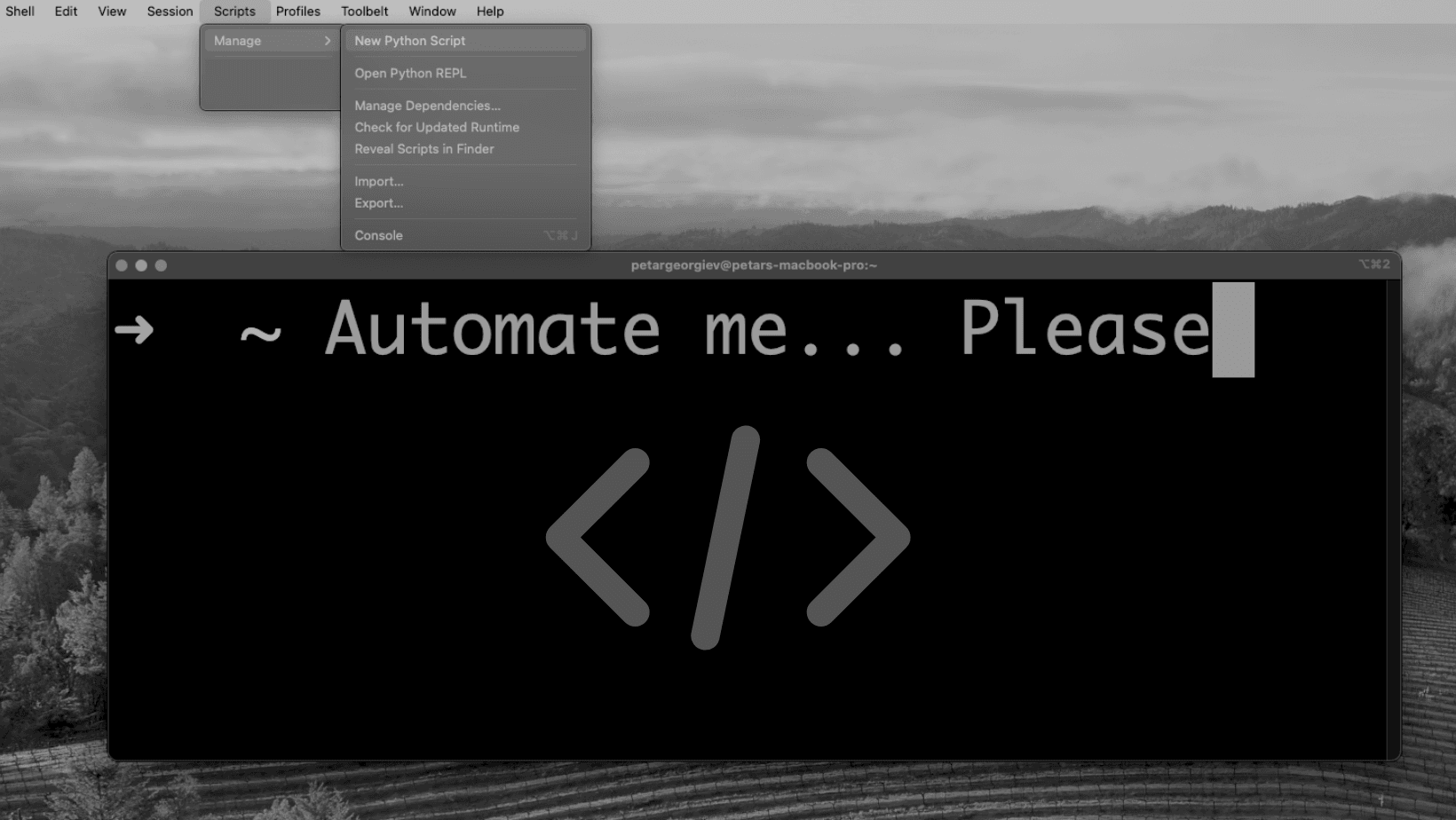
I've spent much time writing different bash scripts or commands to automate my job. An easy but useful script is one to avoid writing cd into a folder 5 times to run first - the database (docker-compose), server, and client. What I've learned is that if you write and do something in a repeated way more than twice you should look into automating it.
What we're going to write is a Python script that will be used with iTerm2. This article assumes that you have already installed iTerm on your machine.
Creating our script
First up, open the iTerm2 application and see the navigation on top of your screen as shown in the photo, and go to Scripts > New Python Script
As you move forward, you will be presented with an option to choose from basic/full environment. If you decide to fully automate a job, you can go with Full Environment, but for our case, we're going with Basic and Simple. When prompted just write the name of the file and the editor of your choice will be opened.
At the time of writing the sample script you're presented is:
#!/usr/bin/env python3.10
import iterm2
# This script was created with the "basic" environment which does not support adding dependencies
# with pip.
async def main(connection):
# Your code goes here. Here's a bit of example code that adds a tab to the current window:
app = await iterm2.async_get_app(connection)
window = app.current_terminal_window
if window is not None:
await window.async_create_tab()
else:
# You can view this message in the script console.
print("No current window")
iterm2.run_until_complete(main)
It's actually a good start. Let's modify it to make it do a couple of things.
- Define the commands that will be triggered in our terminals
- Open a new terminal window
- Open tabs, one by one, and trigger the command that is defined above
#!/usr/bin/env python3.7
import iterm2
# The commands to run
cmd_compose="cd Documents/<path>/ && docker compose up"
cmd_client_run="cd Documents/<path>/ && npm run dev"
cmd_service_run="cd Documents/<path>/ && npm run develop"
async def main(connection):
app = await iterm2.async_get_app(connection)
# Create a new window with the default profile
window = await iterm2.Window.async_create(connection)
if window is not None:
# Start a new tab
tab = window.current_tab
docker_compose_tab = tab.current_session
await docker_compose_tab.async_send_text(cmd_compose + '\n')
await window.async_create_tab()
client_tab = app.current_terminal_window.current_tab.current_session
await client_tab.async_send_text(cmd_client_run + '\n')
await window.async_create_tab()
service_tab = app.current_terminal_window.current_tab.current_session
await service_tab.async_send_text(cmd_service_run + '\n')
else:
# You can view this message in the script console.
print("No current window")
iterm2.run_until_complete(main)
As stated above, we defined our commands and opened tabs in the terminal while executing commands in them. It's actually that simple. Save the file and go again to the navbar, as now you will have your script shown as Script > your-script-name.py. Open it up and it should show all the tabs opened with the corresponding commands executed.
You can create complex flows and scripts that will automate your job and save you time. This is not a one-way solution. How to create and update it is up to you, I've just shown you how to implement a simple script that saves me time daily. If you liked the article you can follow me or just leave a message on Twitter/X.
Related articles
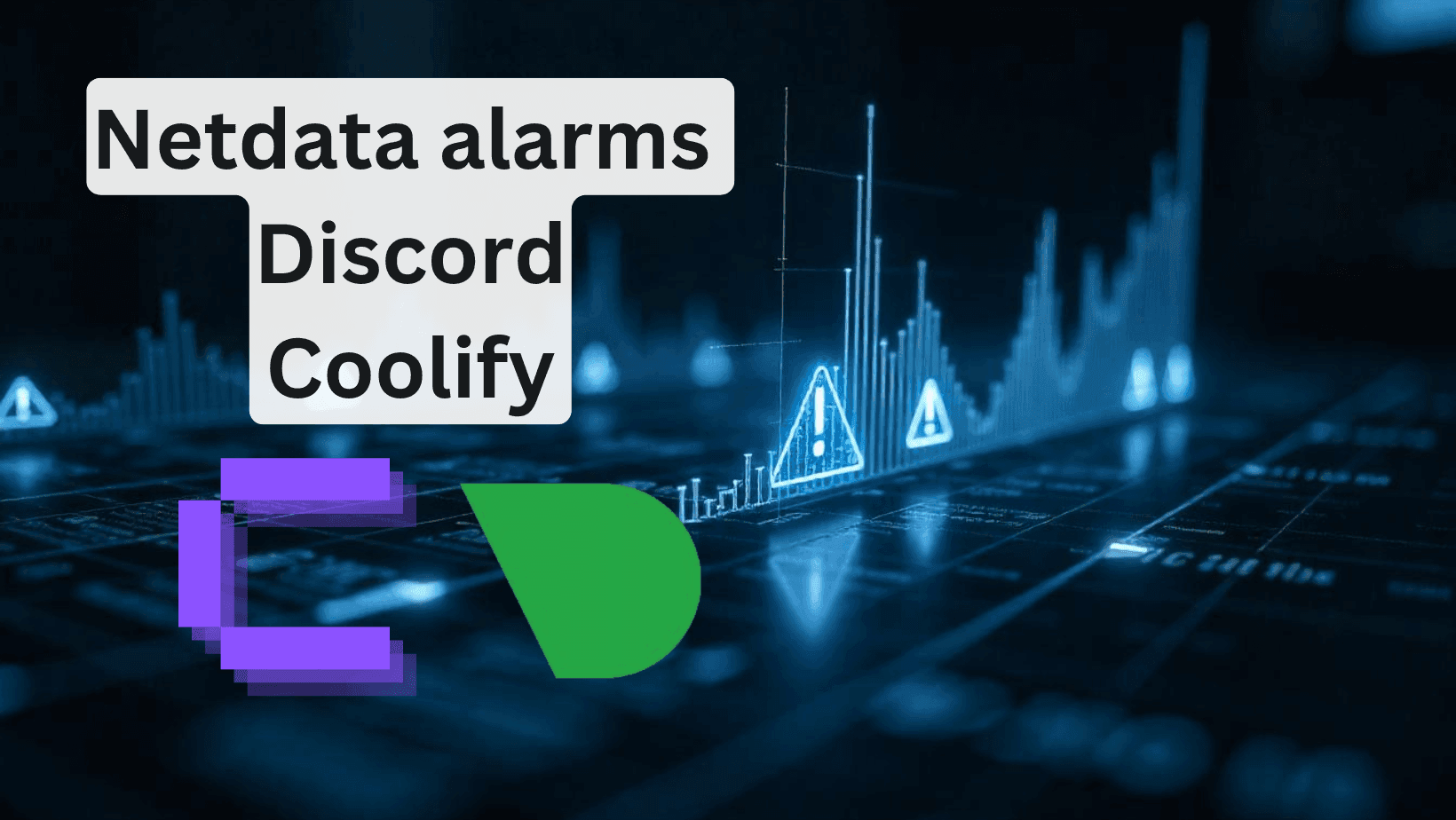
How to add Discord alarms to self-hosted Netdata (Coolify)
Having monitoring without alerts is like having a laptop without internet. It's good to have, but mainly useless.
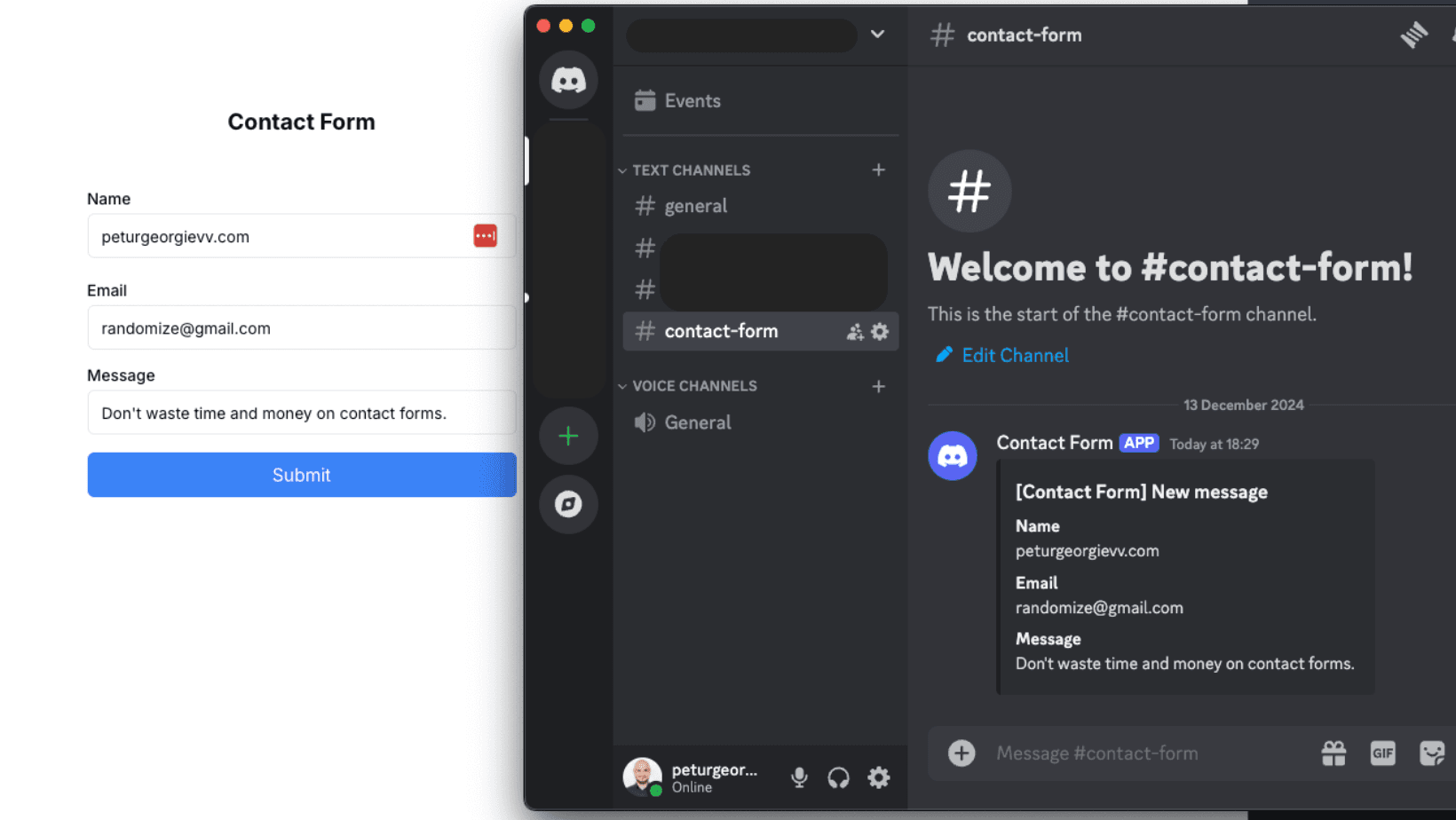
How to Create a Contact Form with Discord and NextJS (FREE)
How to avoid setting up STMP providers and complex email validations and create a discord channel to send contact forms information.
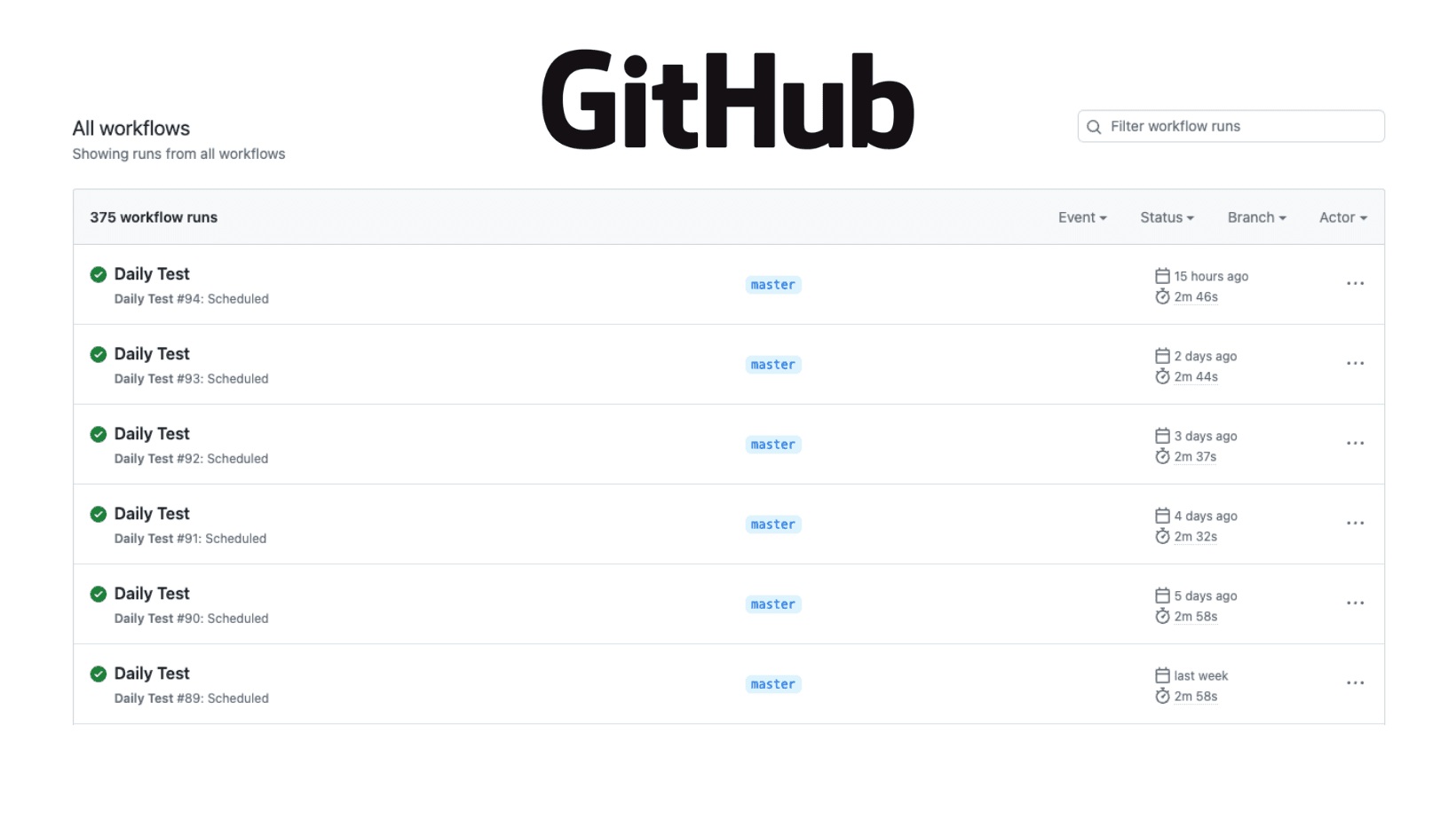
How to Create GitHub Actions - Build & Test your Application on Pull Requests
Create GitHub actions that automate the process of building and testing your application on every pull request to avoid further issues.
My Newsletter
Subscribe to my newsletter and get the latest articles and updates in your inbox!